Here is the code:
int switchPin = 2; // switch input
int motor1Pin = 3; // H-bridge leg 1
int motor2Pin = 4; // H-bridge leg 2
int speedPin = 9; // H-bridge enable pin
int ledPin = 13; //LED
int potPin = 0; //Analogue input from Potentiometer
int potValue = 0; //Value being read from Potentiometer
void setup() {
Serial.begin(9600); //Beggin serial communication
// set the switch as an input:
pinMode(switchPin, INPUT);
// set all the other pins you're using as outputs:
pinMode(motor1Pin, OUTPUT);
pinMode(motor2Pin, OUTPUT);
pinMode(speedPin, OUTPUT);
pinMode(ledPin, OUTPUT);
}
void loop() {
potValue = analogRead(potPin); //Read pot value
Serial.println(potValue); //print value to debugg
// if the switch is high, motor will turn on one direction:
if (digitalRead(switchPin) == HIGH) {
digitalWrite(motor1Pin, LOW); // set leg 1 of the H-bridge low
digitalWrite(motor2Pin, HIGH); // set leg 2 of the H-bridge high
digitalWrite(ledPin, HIGH); //Light up LED for debugging
analogWrite(speedPin, potValue/4); //Write to speed pin to change speed
}
// if the switch is low, motor will turn in the other direction:
else {
digitalWrite(motor1Pin, HIGH); // set leg 1 of the H-bridge high
digitalWrite(motor2Pin, LOW); // set leg 2 of the H-bridge low
analogWrite(speedPin, potValue/4); //Write to speed pin to change speed
blink(ledPin, 3, 100); //Blink LEDs for debugging
}
delay(10); //Delay before next loop
}
/*
blinks an LED
*/
void blink(int whatPin, int howManyTimes, int milliSecs) {
int i = 0;
for ( i = 0; i < howManyTimes; i++) {
digitalWrite(whatPin, HIGH);
delay(milliSecs/2);
digitalWrite(whatPin, LOW);
delay(milliSecs/2);
}
}
Here is the picture of the circuit:
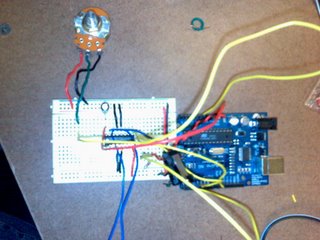
Here is the video of it working:
No comments:
Post a Comment