House Rules
• 10 minutes late – no talking during class
Lesson today – “Enough physics to make a flashlight”
When buying a flashlight you look at:
• Durability
• Light intensity
• What kind of batteries
• How many batteries
• Environmental considerations
• Cost
• Weight
• Cost of upkeep
• Initial investment
• Size
• Rechargeable
• Water proof / floating
• Aesthetics
• Batteries included?
• Brand
• Kind of bulb – durability
• Color of light
• How do direct the light
• All these are design and market niche considerations, unless these were considerations for industrial applications.
What do they all have in common?
• Power source
• Bulb/lamp
• On/off switch
What is different
• Color – appeal / intended audience / preferences / marketing
• Size & shape – how you hold / use it / where – ergonomics
• Texture of material
• Shape of beam
• Brightness
• Physical style of switch – some stay on, some you have to hold to stay on
• Because of these differences you should learn the physical computing side of things.

Terms / jargon on the package we do not recognize:
• LED
• 6V latten battery
• KPR 113 – K13 bulb
• 120V AC
• 2.5 Volts
• 0.3 amps
• 60 Hz
• H14 flashlight bulb
• Krypton bulb
• Polarized
• Adapter
• Watts
Let’s build a flashlight
• Biking headlight – to see or to be seen
• Headlight to work on pComp project w/o waking up roommates
• Laser pointer
• Purse light
Glasses
• Limiting factor – what should we worry about the most?
• Size and weight
• Color
• Switch style
• Bulb
• LED (Light Emitting Diode)
When shopping for light bulb you look for Power/Watts – which do NOT relate to brightness
• Watts = Power = Volts/second
• www.jameco.com - good for browsing
• Opto & illumination
• Lamps – incandescent
Description is size & shape
• It’s light
• It’s small
• It does not heat up
• Consumes less power
• Brightness / Luminous intensity
• MSCP (Mean Spherical Candle Power)
• MCD (Milli candela)

Watts
• Ability to do work
• Energy/time
• E = mc2
• E = force x distance
• Mass ≠ weight
• Weight depends on gravity
• Mass is resistance to change
• m = E / c2
• Coulomb/ second
• Current = amperage = amps = a
• Voltage is like potential / pressure / gravitational pull
Amperage
• How many electrons
Voltage
• How much strength they have
Watts = Volts x Amps
Atoms
• Electrons – valence sheet gardens
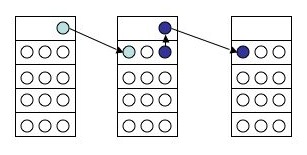
Electricity
• Electrons go from one atom to the other
• Atoms with “vacancies” are good conductress
• Insulators have no/little vacancies
Circuit
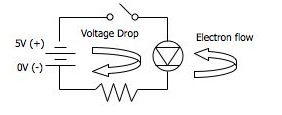
• All LEDs have a flat side – flat goes to 0V (ground)

Bread board (protyping board)
• Put LEDs across different rows
• Voltage regulator
• Takes the voltage down to appropriate value
• Instead of using batteries, plug into wall
• 2 LEDs of 1.7V = 3.4 voltage regulator
• Most microchips use 5 Volts
• IGO – In Ground Out
• Red Line => Voltage
Strip wire 1/4 or 1/8 of inch
• Place on bead board where you want it
• Take out
• Cut / strip / back in

In diagram:
Voltage is 5V, LED is 1,7 - need resistor not to blow it up - what resistance?
-> 5.0 V (power supply) - 1.7 V (LED) = 3.3 V (need to drop ot this current)
-> V = IR => R = V / I => R = 5v / 0.02 a => R = 165 Ω
-> 165Ω would be exact - always put a greater resistance than required - 220Ω would do
• Ohm’s Law => V = IR (Voltage = Current x Resistance (ohms Ω))
• W = IV (Watts = Current x Voltage)
Resitors Color code
• 0 = black
• 1 = brown
• 2 = red
• Poster in the lab
• Nice link:
• http://www.breakup.de/resources/resistor.html
Switches
• All kinds – basically good marketing on two metal pieces touching each other
• ON – closed circuit
• OFF – open circuit
ADMIN STUFF
• We are a week off the other classes
• Friday help sessions are a week ahead
• “Make up” session on the 23rd?
• Read syllabus
• Choose week you do notes on
• Join pComp list serve
• Introduce yourself on the class site
• Sign up for shop cleaning
• Do shop safety
• Look at links
• Think about the light bulb glasses
• Keep journal for class – document your work
• Look for blog with RSS feed – it will feed the wiki
• Read chapters 1 – 3 of Physical Computing book
• Course packet – buy it at NYU book store